Introduction
The word instance in computer science is used with different meanings, for example we can talk about the instance of a computer program to refer to the program that is being executed. In this article we are going to see the concept of INSTANCE in object oriented programming, i.e. the instances that are created from a certain programming CLASS.
What is an INSTANCE in programming
For example, if we have a class called “Cat” some of its properties could be “Name” and “Fur Color”, while some of its functions could be “Eat” and “Meow”.
The Cat class will allow creating Cat type objects and each one of these objects will be an INSTANCE of the Cat class, inside it will have the properties and functions defined in the class, but the information in its fields will be specific to each INSTANCE, that is to say, each object will be an independent instance with its own state, this means that we can have for example two instances of the Cat class each one with a different name and fur color.
Programming objects are abstract entities that exist in the memory of computers, the concept is very useful because it allows to increase the abstraction to solve problems.
Constructors
To create an INSTANCE of a class we use the so-called “Constructors“, functions that allow us to enter the object data and return as a result the reference of the new object.
An example of a Constructor for the Cat class in some programming languages such as Java and C# could be the following:
public Cat(string name,Color color){
this.name = name;
this.furColor = color;
}
Then to create new objects the “new” instruction is used, as shown in the following example:
Cat aCat = new Cat(“Charles”,Color.white);
This will create an object of the Cat class with name “Charles”, white color and the object reference will be stored in the “aCat” field.
Examples of object INSTANCES in Unity
In Unity in most cases we will not need to define constructors for classes or use the “new” instruction, since there are many things that the engine takes care of. In general the Scripts that are created in Unity are MonoBehaviours so Unity is in charge of creating these objects, we simply have to tell it when or where to create them.
We are going to see the concept of Instance in programming applied to the Unity engine with the Cat class example.
Definition of the “Cat” class
We start creating a new Script by right clicking on the Assets folder, going to “Create” and choosing “C# Script”, we name it “Cat”, this Script will define the “Cat” class we talked about before.
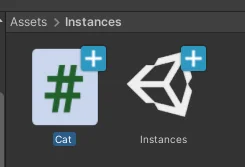
When creating a new Script, Unity is in charge of defining a class with the same name, it will inherit from the MonoBehaviour class and will define some useful functions such as Start and Update, in figure 1.b you can see the Script as it was defined by Unity.
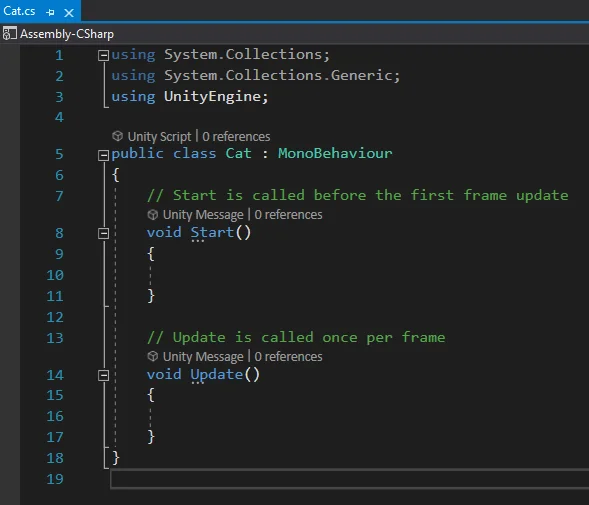
We will modify it as follows:
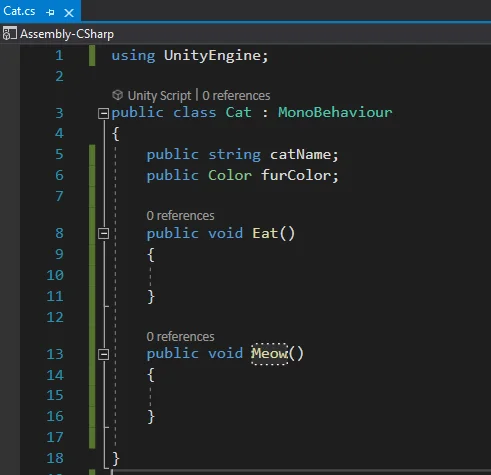
As shown in Figure 2, two fields have been defined, a string called “catName” for the Cat’s name and a Color called “furColor” for the fur color. In addition two functions have been added, Eat() which makes the Cat eat and Meow() makes the Cat meow.
Create INSTANCES of the “Cat” class
The vast majority of Scripts we create in Unity will be similar to this in the sense that they will inherit from the MonoBehaviour class. To create an INSTANCE of this type of objects just add them to some GameObject in the Unity hierarchy or create them using the Instantiate() function.
Let’s start by creating empty objects that will contain the INSTANCES of the Cat class, figure 3 shows how to create an empty GameObject.
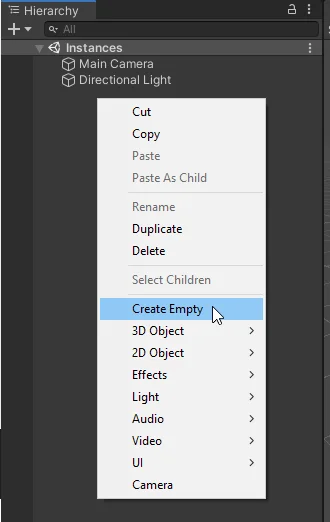
In figure 4 we see two GameObjects, one called Cat1 and the other Cat2, both will have an instance of the same Cat class.
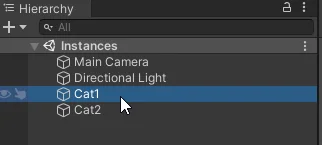
To create the INSTANCES of the Cat class you have to add the Script as a component of the GameObject, this can be done by selecting the GameObject to which you want to add the component and taking the Script from the Assets folder and dragging it to the inspector. Another way to add the component is by using the “Add Component” button that appears in the inspector.
In figures 5 and 6 you can see the inspector of the GameObjects Cat1 and Cat2 of figure 4, as you can see, each one has a component type “Cat”, that is to say, each one has assigned an INSTANCE of the Cat class. Notice also how their fields “catName” and “furColor” in each case have different information, since each INSTANCE represents an individual Cat.
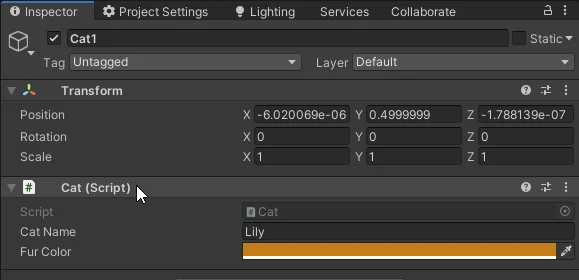
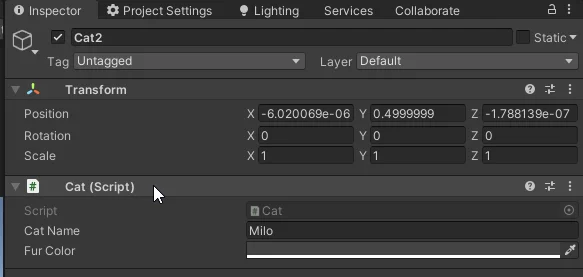
With this we have created two instances of the Cat class, as an extra detail the instances can be assigned to the same GameObject, as shown in figure 7.
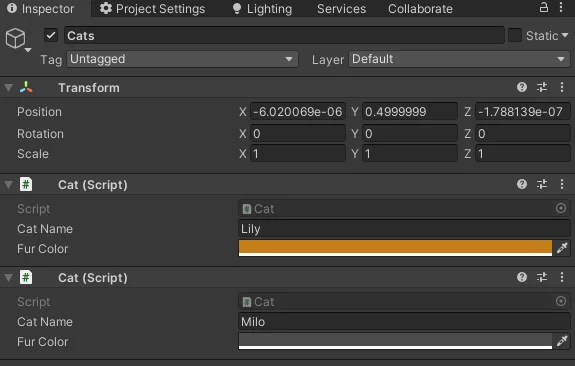