Introduction
In this article we see what is a method in programming, what they are for and how to declare them in C# language with concrete examples in a game project in Unity.
¿What is a Method in Programming?
In this context a method is a function that has a set of instructions defined within it.
The method has a name to identify it.
We can make it require different type of input data to run. And we can make the method return data as a result.
When we need to execute the instructions contained in the method, we do so simply by using its name.
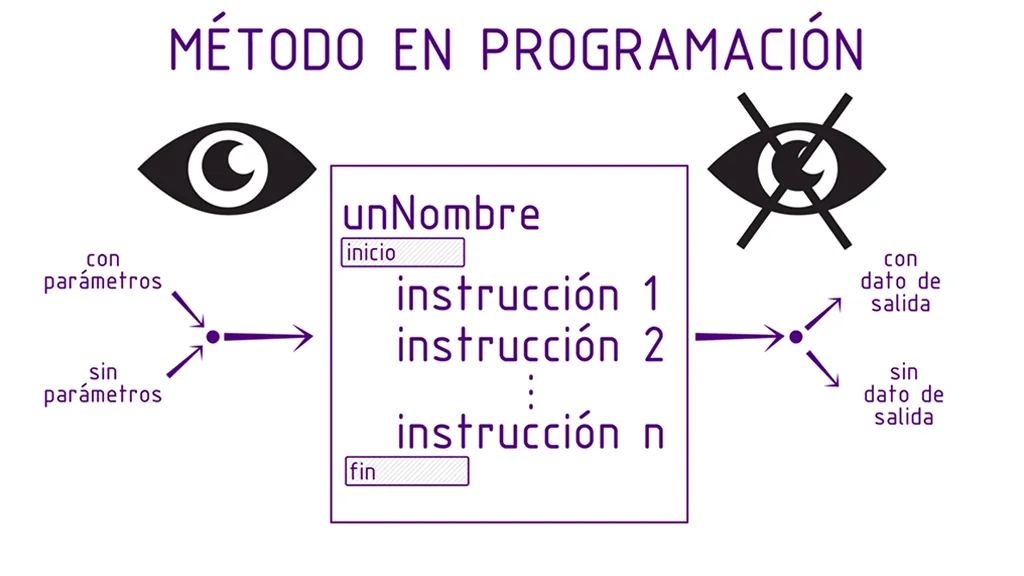
Modularity
In figure 1 we see the method represented as a box, in which the name of the method appears as title, the beginning and end of the method is specified and the set of instructions is within this region.
To understand what modularization is all about, see figure 2. On the left we see a long list of programming instructions.
Now within that list are a number of instructions that are used in more than one place, instructions A, B, C, and D. Let’s say these instructions perform a particular task and are always executed together.
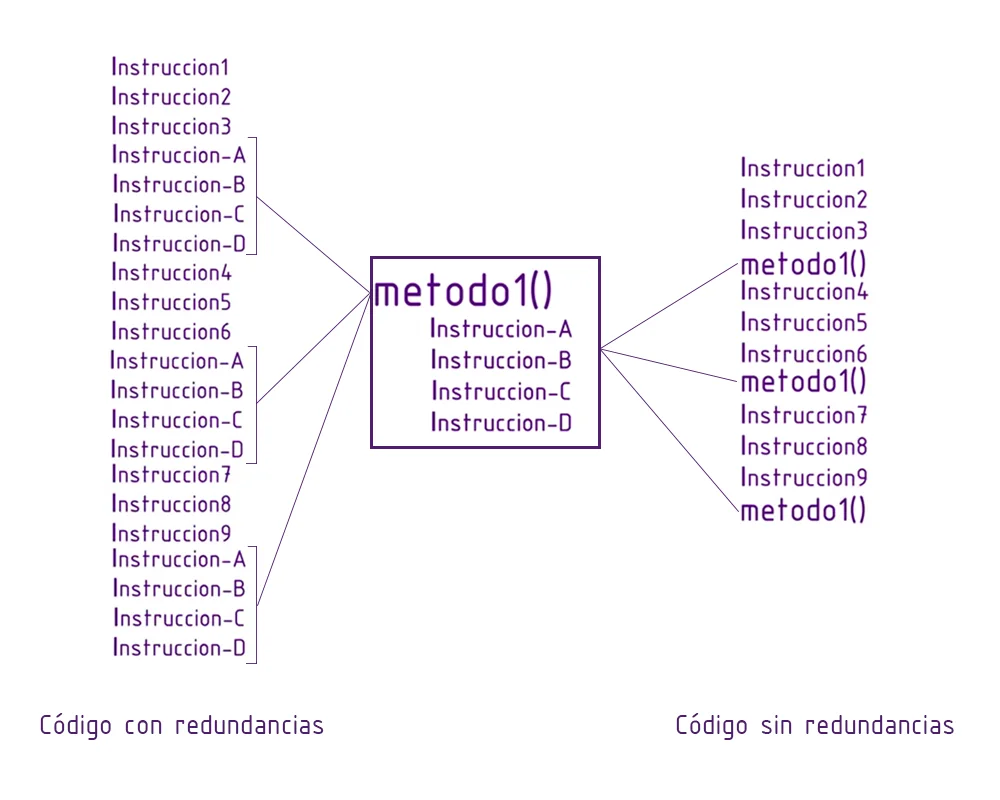
Suppose we now have to make changes to those instructions (for example to improve the way it solves the task or add functionality), what happens is that we have to identify all the regions where those instructions are.
It would be great if we could change things in one place and the changes apply to all regions where these instructions are used, wouldn’t it?
This is precisely what we achieve with the methods, they allow us to group sets of instructions in an independent module that can be called when necessary, as we can see on the right in figure 2, the instructions were now replaced by the name of the method.
Input and Output parameters
In figure 1 there are arrows that go in and out of the method. With this I try to represent the input and output of parameters.
We can make our method require input parameters, which we use inside to make calculations or perform any type of task.
In addition we can make the execution of the method produce an output data, which we will use outside the method.
Public and private methods – Visibility
Finally in figure 1 we see a normal eye and another crossed out eye. This represents the visibility that the method will have, which is an object-oriented programming topic, we are not going to delve into this article but in principle let’s say that we can have public methods and private methods.
Public methods can be executed from other Scripts for example, or we can execute them from a component in the hierarchy in Unity, for example a button.
Private methods will not be accessible from contexts external to the script where it is defined.
Syntax
The syntax is the set of rules established in a language to write the code, in figure 3 we see possible syntax of a method,
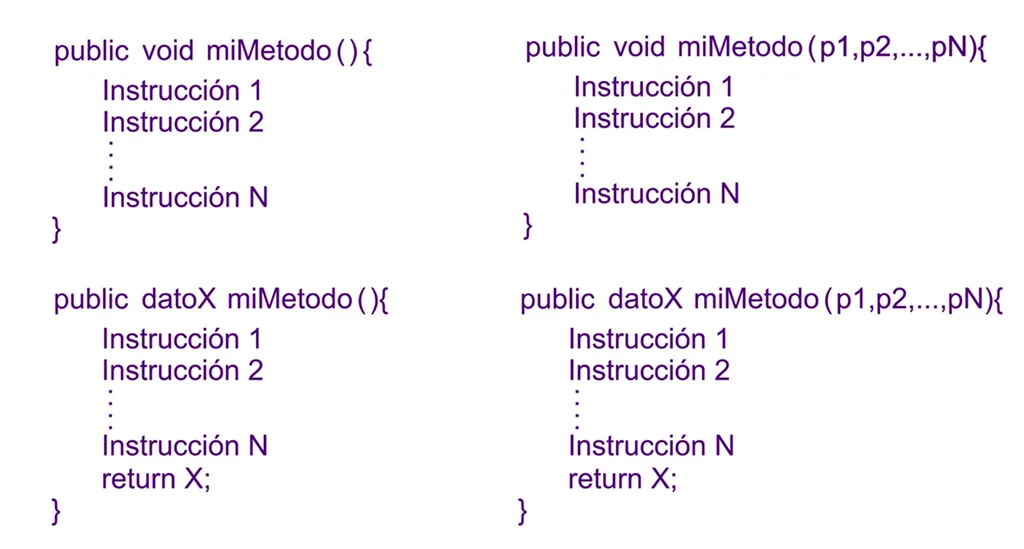
This syntax can be applied to C# and Java, one detail is that all methods are public, but we could replace the word “public” with “private” and the syntax would be correct.
Declaration of a method
Example 1
Let’s analyze the method that is above to the left in figure 3.
We have the structure of a public method that does not require parameters and does not return parameters.
This structure can be found for example in the script “GameControl” of the project My first game in Unity.
We worked on this script in video 4 of the series.
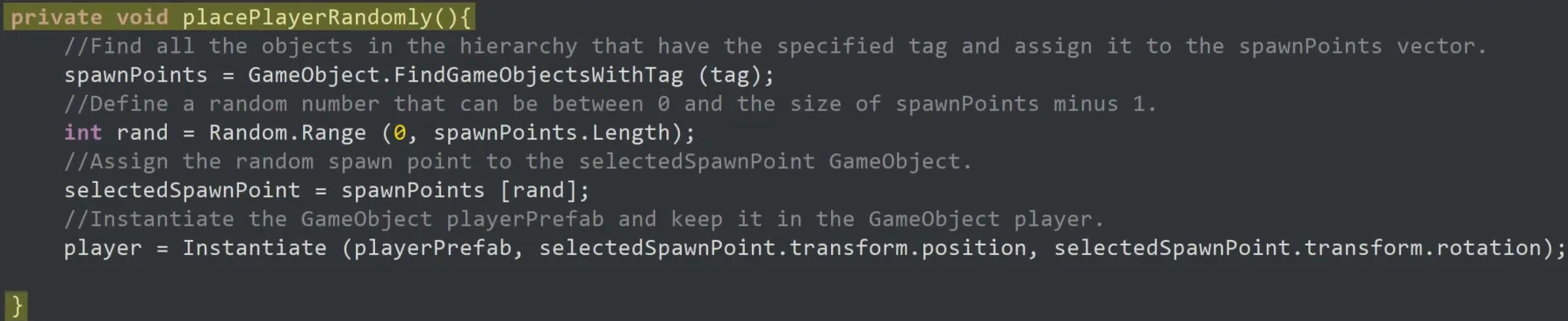
We observe that it has been declared as private instead of public, then we find the word “void” with which we indicate that this method will not return parameters.
Then the name of the method: “placePlayerRandomly”.
We open and close parentheses indicating that this method does not need parameters.
The beginning and end of the method is indicated using keys. Inside will be all method instructions.
Invocation or call to the Method
Once the method is defined we can make it run from any part of the script, we can even invoke it from other scripts.
The following figure shows the invocation of the “placePlayerRandomly” method in the “startGame” method of the same “GameControl” script.
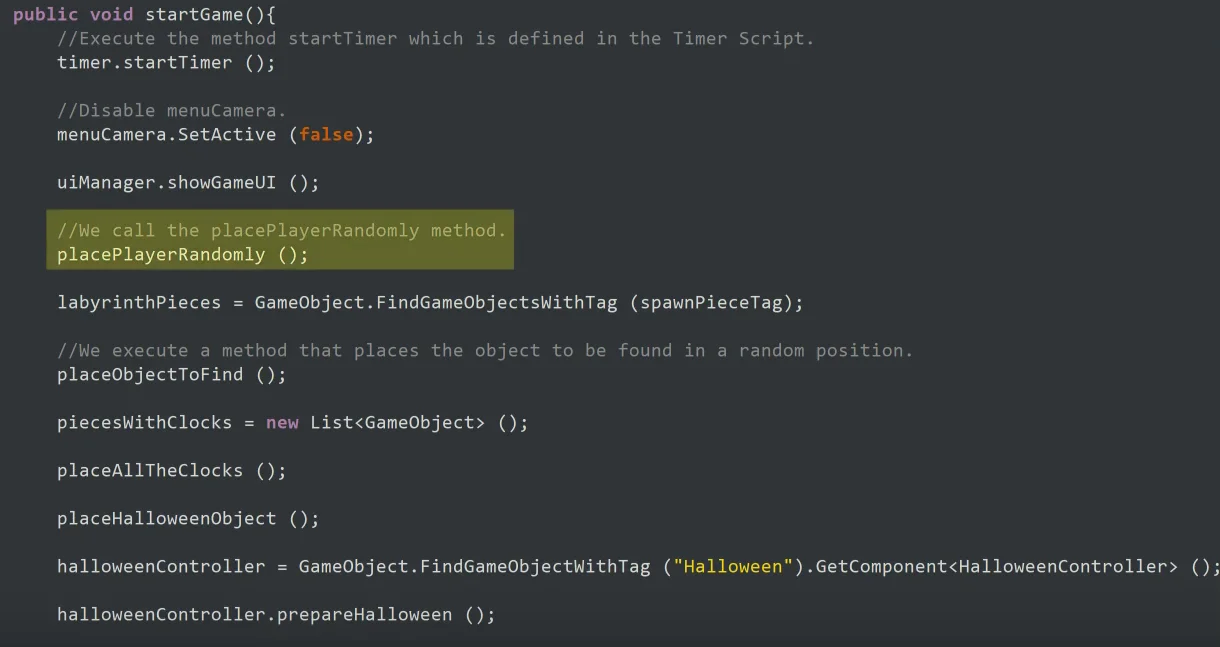
To invoke this method we write its name and open and close parentheses, we close the instruction with semicolon. By doing this we are achieving that all the instructions of the method are executed.
Notice how the names of the methods are already helping us to understand what is going on. At the beginning of the game the character should be placed randomly.
From the “startGame” point of view the “placePlayerRandomly” method is a function that does everything necessary to place the character randomly. The startGame method doesn’t know exactly how it does it but it doesn’t care, because placePlayerRandomly does its job well.
Example 2
In the upper right corner of figure 3 we have the structure of a method that does not return parameters but does require them to work.
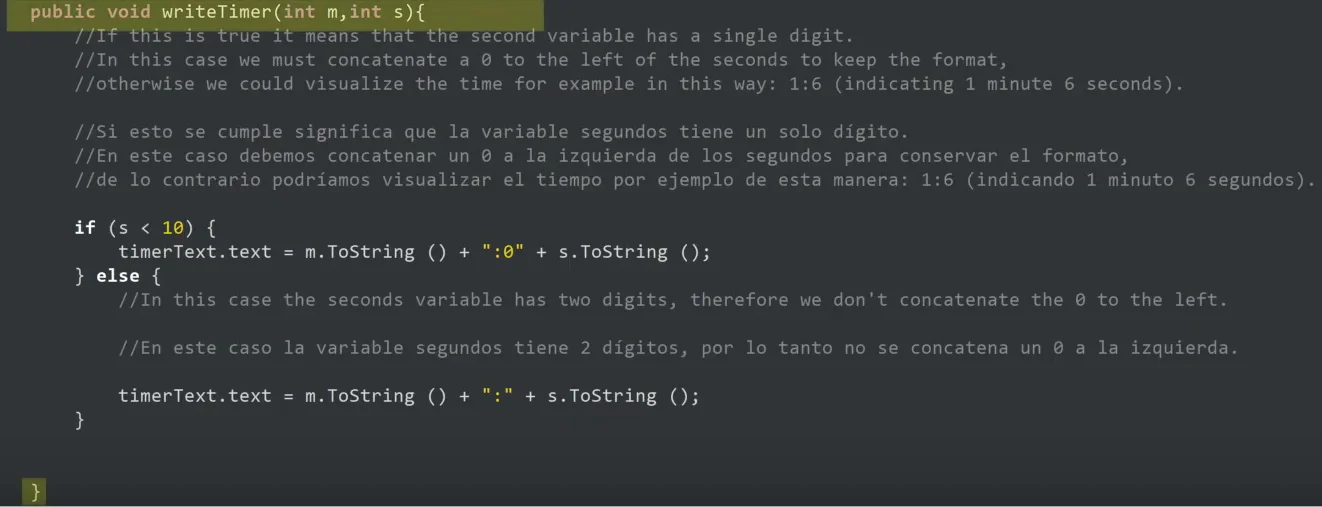
This method requires two parameters to work: an integer value m that will indicate the minutes of the timer and an integer value s that will indicate the seconds.
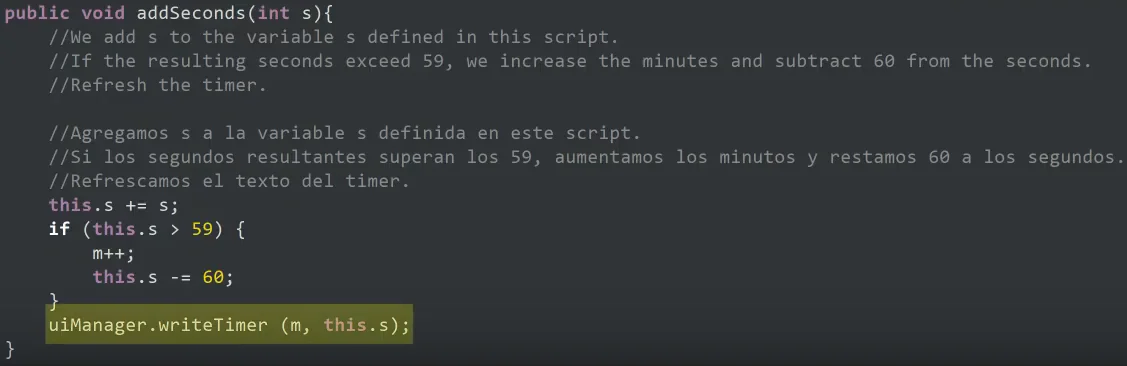
The invocation of this method occurs in several places of the code, in particular the method “addSeconds” belonging to the Script “Timer”, this method receives as parameters an integer “s” that represents the amount of seconds that must be added to the timer because the character grabbed one of the clocks that are on the stage.
The “addSeconds” method does some operations with the “s” variable to update the time and finally executes the “writeTimer” method that shows the new time values on screen.
Two observations: the first is that since we are executing the method from another Script it is necessary to add “uiManager”. (pay attention to the dot) before writing the name of the method “writeTimer”.
The second is that parameters are entered within parentheses and separated by comma.
In both scripts I used confusing names (m and s) on purpose so that in the future, when we talk about contexts, we can analyze them.
Example 3
We move on to methods that return parameters.
The following example corresponds to the structure illustrated in the lower left corner of figure 3, a method that returns data and does not require parameters to function.
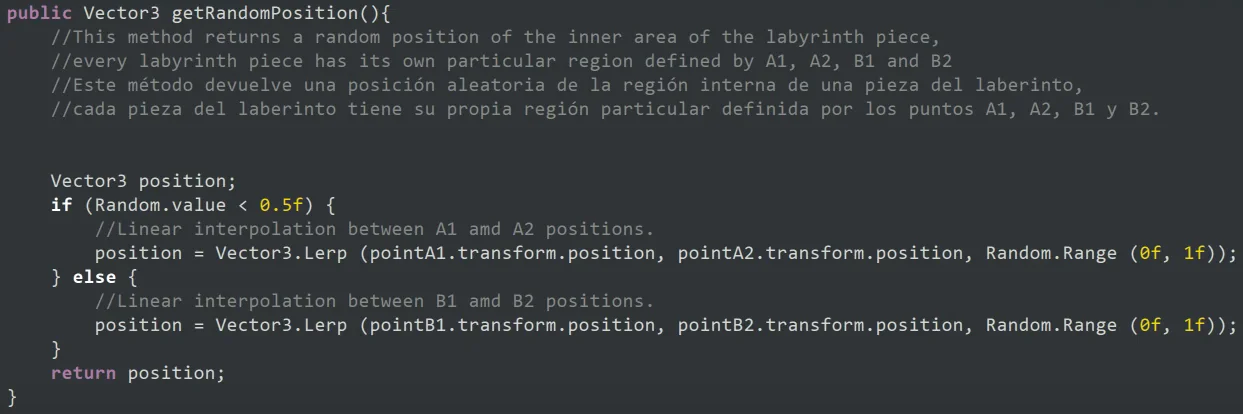
The data returned by this method is a “Vector3” and is indicated in the declaration, after the word “public”. Notice that the first line that is not a comment says: “Vector3 position;”, there we are declaring a Vector3 that we call “position”, then we do some calculations and at the end of the method we write: “return position;” indicating that we return that Vector3.
This method returns a Vector3 (component x,y,z) that represents a random position belonging to the inside of a given piece of labyrinth. In this way we can use this position to place the pedestal or clocks without worrying for example that they are embedded in a wall.
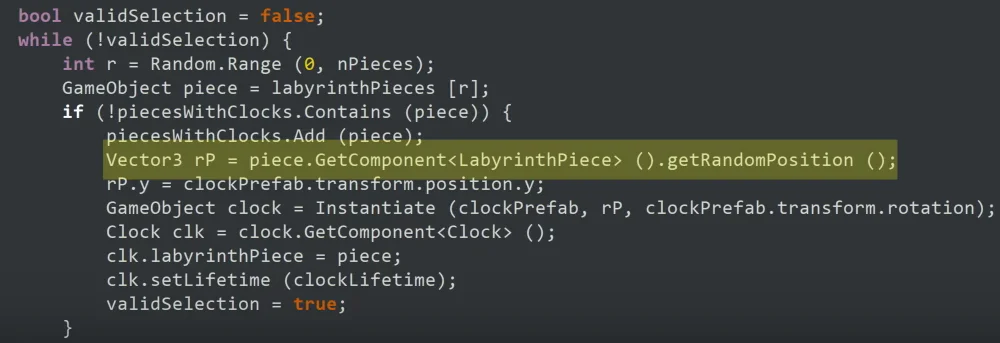
It is not easy to understand the instruction highlighted in figure 9. For now let’s look at two things, the first is that we make a call to the “getRandomPosition” method using its name (as seen at the end of the instruction). The second is that the result of that invocation is a Vector3 data, therefore, using the equal sign, we assign it to the “rP” data that is defined as Vector3.
Example 4
The last example corresponds to the structure illustrated in the lower right corner of figure 3, a method that returns data and requires parameters to function.

We see that in the declaration we say that the method “isBestScore” returns a bool type data and requires an int type data that we call “val”.
If you want to know a little about primitive data I invite you to read this article about variables or watch this video.
The “isBestScore” method returns a variable that can be true or false. The utility of this method is that we use it to ask if the score obtained in the game (val) is better than the one saved so far (bestScoreValue).
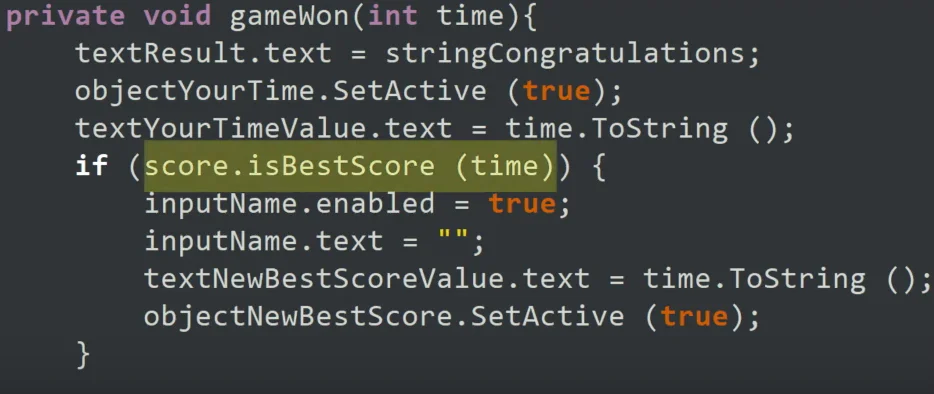
This method is invoked in the “gameWon” method. Since the method returns a bool value, we can use it directly as an argument for an if statement, because “score.isBestScore(time)” is equivalent to having a true or false value.
StartGame() Mehotd from GameControl
To finish figure 12, the “startGame” method is observed along with the other methods it invokes.
In addition to grouping instructions, a method must have a well-established purpose that is consistent with the name we gave it, so that we can quickly know what its function is and what type of instructions it may contain.
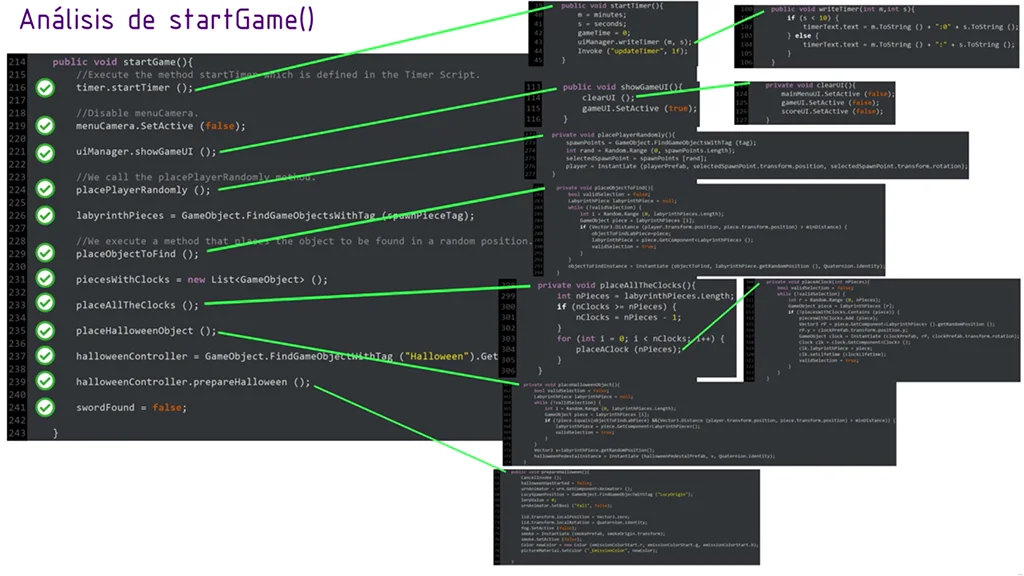
For example, the “startGame” method is in charge of coordinating a series of actions to start the game, but it is not he who directly performs these actions, but invokes other methods with more specific tasks.
This makes it easier for us to make changes to the code and debug errors.
Conclusion
Methods are a powerful tool for developing specific, efficient, reusable and sustainable solutions.
Sustainable in time because at all stages of development we will have to make changes, so we have to be able to remember or quickly understand how our code works.
This is achieved by using descriptive names, documenting our code, and applying the object-oriented paradigm.