Introduction
In this section we are going to talk about algorithms in a wide sense, specifying the characteristics, pseudocode, flow diagrams and finally an example of problem solving, formulating an algorithm, writing the pseudocode, drawing the flow diagram and finally implementing in C# in Unity for the development of videogames.
What is an algorithm?
An algorithm is a finite series of ordered steps that describe the passage from an initial state to a final state of some process.
In general we use algorithms to solve problems, such as performing mathematical calculations or ordering large lists of data.
Given a specific problem, the algorithm that solves it will be a finite series of sequential steps that lead to the resolution of the problem.
Characteristics of an algorithm
An algorithm consists of a finite sequence of steps.
Your instructions must be well defined and unambiguous, i.e. each instruction must be unambiguously interpreted.
The instructions of an algorithm must be ordered sequentially, i.e. they are ordered instructions.
Pseudocode
The pseudocode is a colloquial language description of the algorithm.
In this case we give the algorithm’s instructions the form it will have in the code, but we don’t use syntax of any particular language.
Flowcharts and algorithms
Flowcharts are graphical representations of algorithms.
Using a set of symbols and connecting with arrows you can describe the series of steps that an algorithm describes.
Implementation of a computational algorithm
For the previous steps we do not need to know any programming language. That is to say given a concrete problem, to devise an algorithm that solves it and then to raise a diagram of flow to have a visual representation, all this we can do without knowing how to program and in some way we do it mentally every time we face the problems in the daily life.
To implement the algorithm in a computer program we must know a specific programming language and everything will depend on what we want to do.
Examples: Algorithms in Unity
Let’s give an example of a problem in the field of video game development with Unity.
Suppose we have a series of enemies surrounding the player and we want to know which of them is the closest.
Presenting the problem
The objective is to create a function or method that, given a set of GameObjects, returns the GameObject that is closest to the player.
Algorithm
The sequence of steps to solve this problem would be:
-Assume that the first element of the set is the one closest to the player.
-For items from position 2 onwards compare them with the closest object so far, if it is even closer to the player take it as the new object to compare. Repeat for all remaining items.
Pseudocode
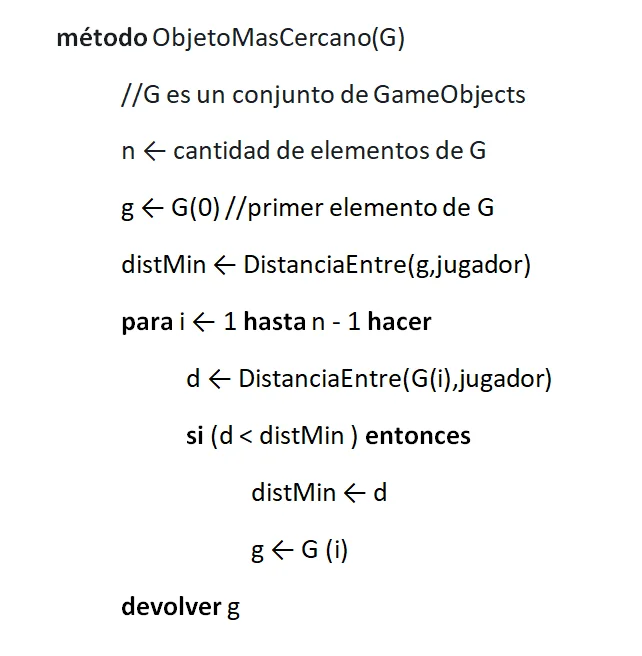
To write the pseudocode we take the algorithm and give it a programming structure in colloquial language, taking into account the assignments, operations, loops, if sentences and so on.
Flowchart
The flowchart will be a visual representation of the pseudocode, using symbols predefined by convention. Below we can see the flowchart associated with the previous pseudocode.
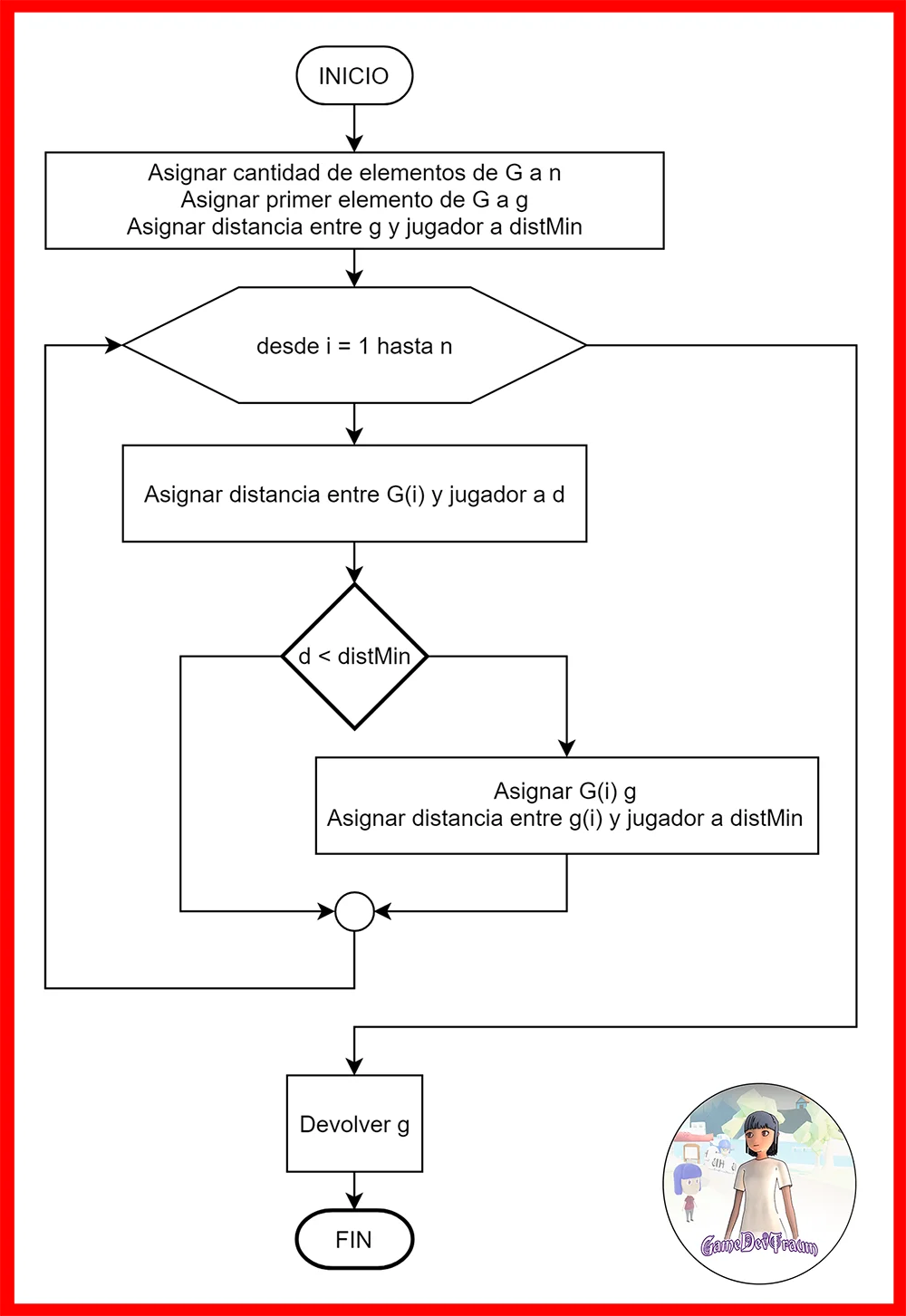
Implementation of the algorithm in Unity
In this part we go to the concrete application of the algorithm, as we are going to use it for a function in Unity (videogame development) the implementation will be in C# language.
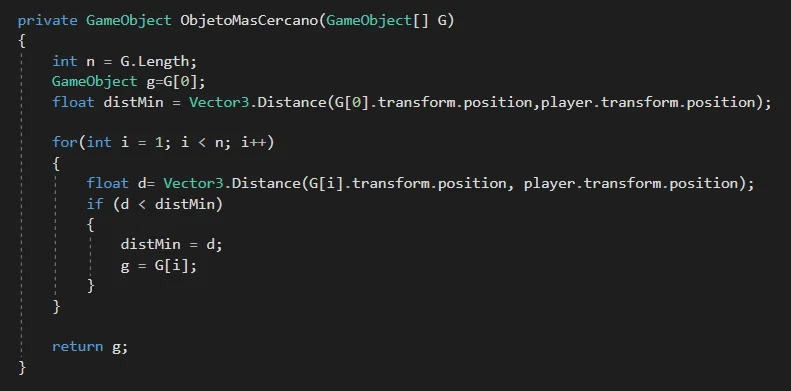
As we can see, the final result is a method in C# that solves the proposed problem.