Introduction – What we are going to do and why
In this article we are going to see a method that will allow us to find a COMPONENT of a specific type in Unity from a Script, for example to find a component type AudioSource, Collider or even a Script that we have created and assigned to a GameObject. The purpose of that is to have the reference of that component inside our Script and to be able to use it according to our needs. For example if we want to call a function that is defined in another Script we are going to need the reference of the instance of that other Script to be able to do it.
The method that we are going to see consists of using a code instruction that checks each GameObject of the hierarchy and its components until it finds the component of the type that we indicate, if there is a component of that type present in the scene the instruction will return it as the execution result and we will be able to store that component in a variable or to use it immediately to execute some simple action on the component that we found. If on the other hand there is no component of that type in any GameObject of the scene, the instruction will return Null and that could lead to an error of type NullReferenceException.
All the IMPORTANT information is summarized in the following TUTORIAL FROM MY YOUTUBE CHANNEL
When is this method useful?
- This method is effective when the component we are looking for is unique in the scene, if there is more than one component of the same type there is no guarantee of finding precisely the one we are looking for.
- It is recommended to use this method in some initialization function like Awake or Start.
- It is not recommended to use this method inside update functions like Update because they imply to traverse in the worst case the whole hierarchy of the scene. Unless the program flow is somehow controlled so that the instruction is executed only once.
- If the component you want to find is going to be used more than once, it is advisable to find it once and store it in a global variable so that it can be used as many times as necessary.
Detailed procedure of finding a component from the scene through code
Initial conditions
We start from a Script called “FindReferenceOfAnObject” in which we are going to find the reference of a certain GameObject that is in the scene in Unity, inside the Script we will use that reference to print its name in console with the instruction of the line 13 of the figure 1.
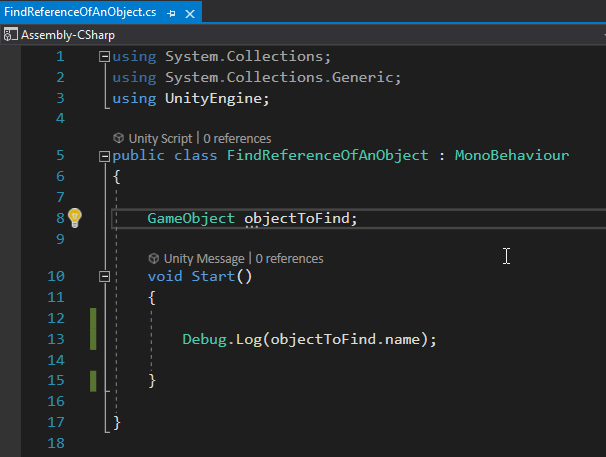
The hierarchy of the scene that we are going to use is composed by the GameObjects that are shown in figure 2, the object “Script-GameObject” is the one that has the Script in figure 1 assigned and it is the one that will be in charge of finding the references, in figure 3 you can see the inspector of this GameObject, where the Script is assigned.
The “GDT (Object to Find)” object seen in figure 2 is the object we want to find from the script, so if we succeed we should see the name of this object printed on the console.
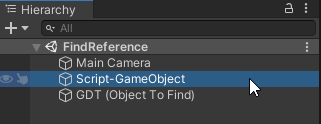
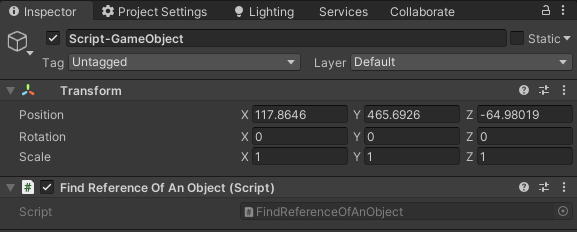
Find the reference of a GameObject that has a specific component
If we know that the GameObject we are interested in finding has a specific component assigned to it, such as a “Camera”, “Rigidbody”, “AudioSource” component or a script we have created ourselves, we can use that knowledge to find the GameObject reference.
I will create a script called “SomeScript” and assign it to the GameObject we want to find, as shown in figures 4 and 5.
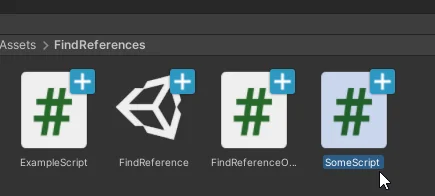
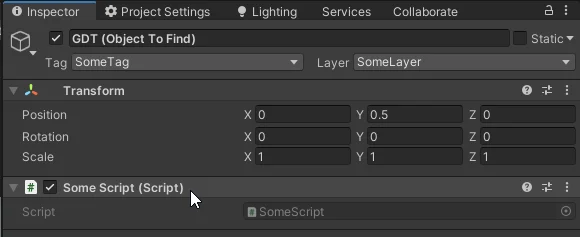
Using the instruction “FindObjectOfType<T>()”, where T is the type of object we are looking for (in our case it is “SomeScript” type), we can find the reference of the “SomeScript” instance that is assigned to that GameObject, then using the dot operator we can access the GameObject to which that Script is assigned.
The instruction that does all this can be seen in line 20 of figure 6.
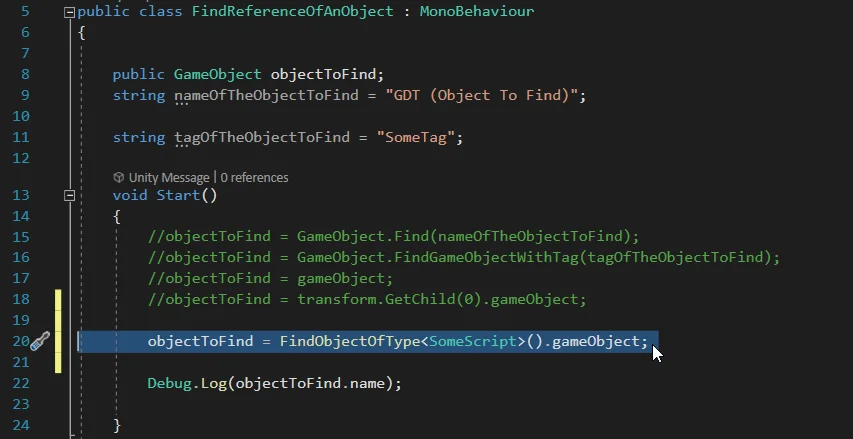
When this instruction is executed, Unity will check all the objects in the hierarchy and each one of its components until it finds a “SomeScript” type object, when it finds it it returns it as a result, but since we are interested in the GameObject to which that Script is assigned, we use the dot operator and access the “gameObject” field. If there is no object that has the “SomeScript” component assigned to it we will have a null reference error because we are using the “objectToFind” field in line 22 of figure 6.
To keep in mind, if we have more than one GameObject that has assigned the component we are looking for, Unity will return the first component that it finds in its register, in this case ambiguities could arise, we could obtain the reference of a different object to the one we want.